How to Create Progressive Web Apps (PWA)
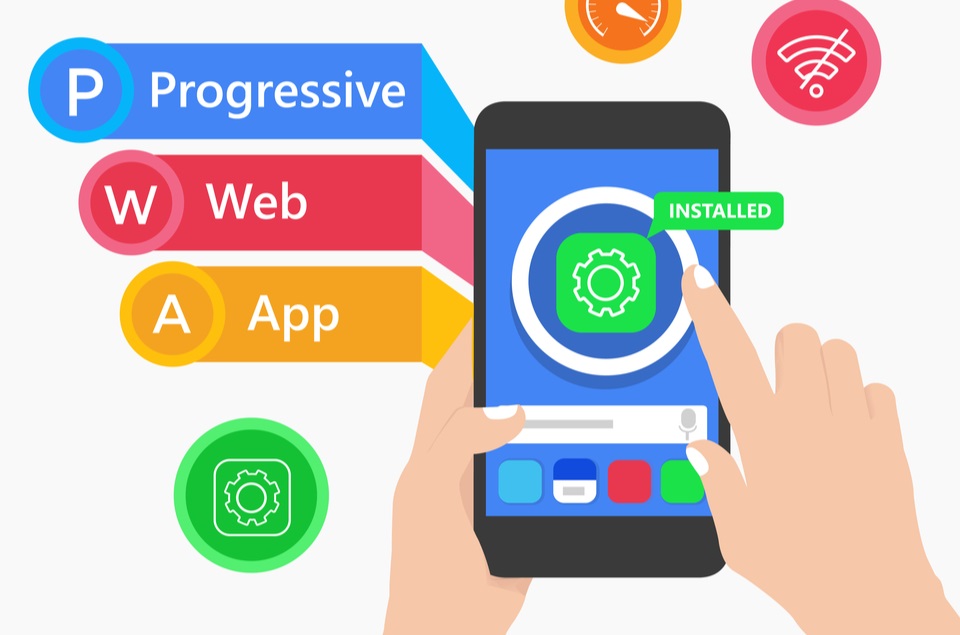
Progressive Web Apps (PWAs) are web applications that offer a native app-like experience on the web. They are designed to work on any browser that complies with web standards, making them accessible to a wide range of users. PWAs combine the best features of web and mobile apps, such as offline capabilities, push notifications, and fast loading times. This comprehensive guide will walk you through the steps to create a Progressive Web App from scratch, covering everything from the basics to advanced features.
1. Introduction to PWAs
1.1 What is a PWA?
A Progressive Web App is a type of application software delivered through the web, built using common web technologies including HTML, CSS, and JavaScript. They are intended to work on any platform that uses a standards-compliant browser, including both desktop and mobile devices. Key characteristics of PWAs include:
- Responsive: Fits any form factor, desktop, mobile, tablet, or whatever is next.
- Connectivity independent: Works offline or with low-quality networks.
- App-like: Feels like an app to the user with app-style interactions and navigation.
- Fresh: Always up-to-date thanks to the service worker update process.
- Safe: Served via HTTPS to prevent snooping and ensure content hasn’t been tampered with.
- Discoverable: Identifiable as an “application” thanks to W3C manifests and service worker registration scope allowing search engines to find them.
- Re-engageable: Makes re-engagement easy through features like push notifications.
- Installable: Allows users to keep apps they find most useful on their home screen without the hassle of an app store.
- Linkable: Easily shared via URL and do not require complex installation.
1.2 Benefits of PWAs
- Improved Performance: With the help of service workers, PWAs can cache resources and deliver fast experiences.
- Offline Support: Users can access content even when they are offline or on low-quality networks.
- Native App Feel: PWAs can be added to the home screen and run in a standalone window, providing a native-like experience.
- Push Notifications: PWAs can engage users with timely push notifications.
- Reduced Development Costs: PWAs use a single codebase for both web and mobile, reducing the development time and costs.
2. Setting Up Your Development Environment
2.1 Prerequisites
Before you start developing a PWA, make sure you have the following tools installed:
- Node.js and npm: Node.js is a JavaScript runtime, and npm is the package manager for Node.js. You can download and install them from nodejs.org.
- Code Editor: A code editor like Visual Studio Code or Sublime Text.
2.2 Project Setup
- Initialize a new project:sh
mkdir my-pwa
cd my-pwa
npm init -y
- Install development dependencies:sh
npm install --save-dev lite-server
- Add scripts to
package.json
:json"scripts": {
"start": "lite-server"
}
- Create a basic file structure:sh
mkdir src
cd src
touch index.html app.js style.css
2.3 Basic HTML Structure
Create a basic HTML structure in index.html
:
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My PWA</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>Hello, PWA!</h1>
<script src="app.js"></script>
</body>
</html>
2.4 Serving the Application
To serve the application, run the following command:
npm start
This will start a local server and open your application in the browser.
3. Adding PWA Features
3.1 Creating a Manifest File
The manifest file provides information about your application in a JSON file. It is used by the browser to install the app on the user’s device.
Create a manifest.json
file in the src
directory:
{
"name": "My PWA",
"short_name": "PWA",
"start_url": ".",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"description": "A simple PWA example",
"icons": [
{
"src": "icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
Link the manifest file in your index.html
:
<link rel="manifest" href="manifest.json">
3.2 Adding Icons
Create icons of different sizes and save them in the src
directory. Update the manifest.json
file with the appropriate icon paths.
3.3 Service Workers
Service workers are scripts that run in the background, enabling features like offline support, background sync, and push notifications.
- Register the service worker in
app.js
:javascriptif ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/service-worker.js')
.then(registration => {
console.log('Service Worker registered with scope:', registration.scope);
}).catch(error => {
console.log('Service Worker registration failed:', error);
});
});
}
- Create the service worker: Create a
service-worker.js
file in thesrc
directory:javascriptconst CACHE_NAME = 'my-pwa-cache-v1';
const urlsToCache = [
'/',
'/index.html',
'/style.css',
'/app.js',
'/manifest.json',
'/icon-192x192.png',
'/icon-512x512.png'
];self.addEventListener('install', event => {
event.waitUntil(
caches.open(CACHE_NAME)
.then(cache => {
return cache.addAll(urlsToCache);
})
);
});self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request)
.then(response => {
return response || fetch(event.request);
})
);
});self.addEventListener('activate', event => {
const cacheWhitelist = [CACHE_NAME];
event.waitUntil(
caches.keys().then(cacheNames => {
return Promise.all(
cacheNames.map(cacheName => {
if (cacheWhitelist.indexOf(cacheName) === -1) {
return caches.delete(cacheName);
}
})
);
})
);
});
- Update the service worker registration in
app.js
:javascriptif ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/service-worker.js')
.then(registration => {
console.log('Service Worker registered with scope:', registration.scope);
}).catch(error => {
console.log('Service Worker registration failed:', error);
});
});
}
3.4 Testing Offline Capabilities
To test the offline capabilities:
- Open the Chrome DevTools (F12).
- Go to the “Application” tab.
- Under the “Service Workers” section, check the “Offline” box.
- Refresh the page to see the PWA load offline.
3.5 Adding a Web App Manifest
The web app manifest provides metadata about the app and helps it be installed on a user’s home screen.
Create a manifest.json
file in the src
directory:
{
"name": "My PWA",
"short_name": "PWA",
"start_url": ".",
"display": "standalone",
"background_color": "#ffffff",
"theme_color": "#000000",
"description": "A simple PWA example",
"icons": [
{
"src": "icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
Link the manifest file in your index.html
:
<link rel="manifest" href="manifest.json">
4. Enhancing Your PWA
4.1 Push Notifications
Push notifications allow you to engage users with timely updates.
- Install the Firebase SDK:sh
npm install firebase
- Initialize Firebase in
app.js
:javascriptimport firebase from 'firebase/app';
import 'firebase/messaging';const firebaseConfig = {
apiKey: 'YOUR_API_KEY',
authDomain: 'YOUR_AUTH_DOMAIN',
projectId: 'YOUR_PROJECT_ID',
storageBucket: 'YOUR_STORAGE_BUCKET',
messagingSenderId: 'YOUR_MESSAGING_SENDER_ID',
appId: 'YOUR_APP_ID'
};firebase.initializeApp(firebaseConfig);
const messaging = firebase.messaging();
messaging.requestPermission()
.then(() => {
return messaging.getToken();
})
.then(token => {
console.log('FCM Token:', token);
})
.catch(error => {
console.log('Error getting permission or token:', error);
});
- Handle Push Notifications:javascript
messaging.onMessage(payload => {
console.log('Message received:', payload);
// Customize notification here
const notificationTitle = payload.notification.title;
const notificationOptions = {
body: payload.notification.body,
icon: payload.notification.icon
};self.registration.showNotification(notificationTitle, notificationOptions);
});
4.2 Background Sync
Background sync allows your app to defer actions until the user has a stable connection.
- Register a sync event in your service worker (
service-worker.js
):javascriptself.addEventListener('sync', event => {
if (event.tag === 'syncData') {
event.waitUntil(syncData());
}
});function syncData() {
// Perform data sync here
return fetch('/sync-data').then(response => {
return response.json();
}).then(data => {
console.log('Data synced:', data);
}).catch(error => {
console.error('Error syncing data:', error);
});
}
- Request a sync in your main JavaScript file (
app.js
):javascriptnavigator.serviceWorker.ready.then(registration => {
return registration.sync.register('syncData');
}).catch(error => {
console.error('Error registering sync:', error);
});
5. Deployment
5.1 Hosting on Firebase
Firebase Hosting is a great option for deploying PWAs.
- Install Firebase CLI:sh
npm install -g firebase-tools
- Initialize Firebase:sh
firebase login
firebase init
- Deploy to Firebase:sh
firebase deploy
5.2 Other Hosting Options
- GitHub Pages: Host your PWA for free using GitHub Pages.
- Netlify: A popular choice for hosting static websites, including PWAs.
- Vercel: Another excellent option for deploying PWAs with minimal configuration.
6. Best Practices for PWAs
6.1 Performance Optimization
- Lazy Loading: Load resources as needed to improve initial load time.
- Code Splitting: Break your JavaScript bundle into smaller chunks.
- Caching Strategies: Use service workers to cache assets efficiently.
6.2 Security Considerations
- HTTPS: Serve your PWA over HTTPS to ensure security and integrity.
- Content Security Policy (CSP): Implement CSP to protect against XSS attacks.
- Service Worker Security: Ensure your service worker scripts are secure and tamper-proof.
6.3 User Experience
- Responsive Design: Ensure your PWA looks great on all devices.
- Offline Experience: Provide meaningful offline experiences, such as cached content or offline pages.
- Fast and Smooth: Aim for a fast, smooth, and app-like user experience.
7. Conclusion
Creating a Progressive Web App involves combining the best features of web and native apps to deliver a seamless user experience. By following the steps outlined in this guide, you can build a robust PWA that is fast, reliable, and engaging. From setting up your development environment to deploying your app, this guide covers everything you need to know to get started with PWAs. Embrace the power of Progressive Web Apps and take your web development skills to the next level.