How to Use asyncio for Asynchronous Programming in Python
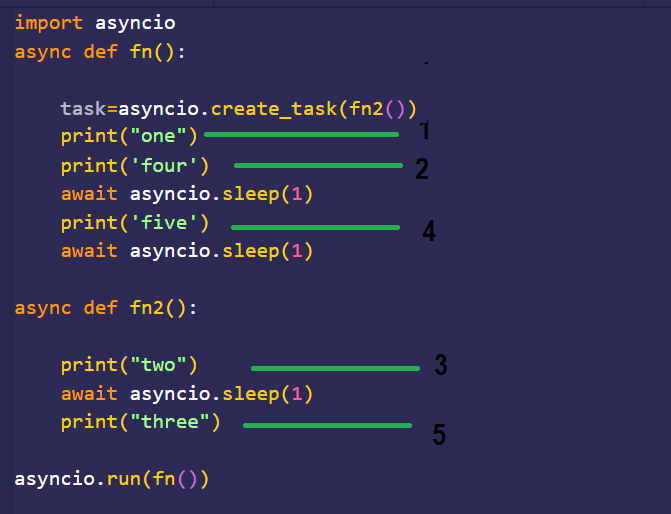
Asynchronous programming is a paradigm that allows programs to perform multiple tasks concurrently, improving efficiency and responsiveness. Python’s asyncio
library provides a framework for writing asynchronous code, making it easier to handle I/O-bound operations and manage concurrency. This comprehensive guide covers the essentials of asyncio
, including its core concepts, practical usage, advanced features, and best practices.
Table of Contents
- Introduction to Asynchronous Programming
- Understanding
asyncio
- Getting Started with
asyncio
- Core Concepts of
asyncio
- Writing Asynchronous Functions
- Task Management
- Using
asyncio
for I/O Operations - Working with Coroutines
- Error Handling in Asynchronous Code
- Synchronization Primitives
- Using
asyncio
with Third-Party Libraries - Best Practices and Optimization
- Debugging and Testing
- Real-World Examples
- Conclusion
1. Introduction to Asynchronous Programming
What is Asynchronous Programming?
Asynchronous programming allows a program to perform other tasks while waiting for operations to complete, such as I/O operations or network requests. This is achieved through non-blocking operations, where tasks can run concurrently without waiting for others to finish.
Benefits of Asynchronous Programming
- Improved Performance: Handles multiple I/O-bound operations concurrently, improving efficiency.
- Responsiveness: Keeps applications responsive by not blocking the main thread.
- Scalability: Handles large numbers of simultaneous connections or tasks with lower resource consumption.
2. Understanding asyncio
What is asyncio
?
asyncio
is a Python library designed for writing asynchronous programs using async
and await
syntax. It provides an event loop, which manages the execution of asynchronous tasks and coroutines.
Key Features of asyncio
- Event Loop: Manages and schedules tasks.
- Coroutines: Functions that use
async
andawait
to handle asynchronous operations. - Tasks: Represent asynchronous operations managed by the event loop.
- Futures: Objects that represent the result of an asynchronous operation that hasn’t completed yet.
3. Getting Started with asyncio
Installing asyncio
asyncio
is part of Python’s standard library starting from Python 3.3, so no additional installation is needed. Ensure you have Python 3.3 or later installed.
Basic Structure of an asyncio
Program
import asyncioasync def main():
print("Hello")
await asyncio.sleep(1)
print("World")
asyncio.run(main())
async def
: Defines a coroutine.await
: Pauses the coroutine until the awaited task is complete.asyncio.run()
: Runs the main coroutine and manages the event loop.
4. Core Concepts of asyncio
Event Loop
The event loop is the core of asyncio
, responsible for executing coroutines and managing tasks.
import asyncioloop = asyncio.get_event_loop()
async def hello_world():
print("Hello")
await asyncio.sleep(1)
print("World")
loop.run_until_complete(hello_world())
Coroutines
Coroutines are special functions defined with async def
and can use await
to pause their execution.
import asyncioasync def coroutine_example():
print("Start")
await asyncio.sleep(2)
print("End")
Tasks
Tasks are used to schedule coroutines for execution. They are created using asyncio.create_task()
or loop.create_task()
.
import asyncioasync def task_example():
print("Task running")
await asyncio.sleep(1)
print("Task complete")
async def main():
task = asyncio.create_task(task_example())
await task
asyncio.run(main())
Futures
Futures represent values that are not yet available. They can be used to track the completion of asynchronous operations.
import asyncioasync def future_example():
future = asyncio.Future()
await asyncio.sleep(1)
future.set_result("Future result")
result = await future
print(result)
asyncio.run(future_example())
5. Writing Asynchronous Functions
Defining Coroutines
Coroutines are defined using async def
and can be paused with await
.
async def fetch_data():
await asyncio.sleep(2)
return "Data fetched"
Using await
to Pause Execution
await
pauses the coroutine until the awaited coroutine completes.
async def main():
print("Start")
result = await fetch_data()
print(result)asyncio.run(main())
6. Task Management
Creating and Managing Tasks
Tasks are used to run coroutines concurrently. They can be created with asyncio.create_task()
or loop.create_task()
.
import asyncioasync def task1():
await asyncio.sleep(1)
print("Task 1 complete")
async def task2():
await asyncio.sleep(2)
print("Task 2 complete")
async def main():
t1 = asyncio.create_task(task1())
t2 = asyncio.create_task(task2())
await t1
await t2
asyncio.run(main())
Waiting for Multiple Tasks
Use await asyncio.gather()
to run multiple tasks concurrently and wait for their completion.
import asyncioasync def task1():
await asyncio.sleep(1)
print("Task 1 complete")
async def task2():
await asyncio.sleep(2)
print("Task 2 complete")
async def main():
await asyncio.gather(task1(), task2())
asyncio.run(main())
7. Using asyncio
for I/O Operations
Asynchronous File I/O
The aiofiles
library provides asynchronous file I/O operations. Install it with pip install aiofiles
.
import aiofiles
import asyncioasync def read_file():
async with aiofiles.open('file.txt', mode='r') as f:
contents = await f.read()
print(contents)
asyncio.run(read_file())
Asynchronous Network Operations
Use asyncio
for asynchronous network operations, such as TCP/UDP communication.
Asynchronous TCP Client:
import asyncioasync def tcp_client():
reader, writer = await asyncio.open_connection('localhost', 8888)
writer.write(b'Hello, Server!')
await writer.drain()
data = await reader.read(100)
print(f"Received: {data.decode()}")
writer.close()
await writer.wait_closed()
asyncio.run(tcp_client())
Asynchronous TCP Server:
import asyncioasync def handle_client(reader, writer):
data = await reader.read(100)
writer.write(data)
await writer.drain()
writer.close()
async def main():
server = await asyncio.start_server(handle_client, '127.0.0.1', 8888)
async with server:
await server.serve_forever()
asyncio.run(main())
8. Working with Coroutines
Chaining Coroutines
Coroutines can call other coroutines using await
.
import asyncioasync def step1():
await asyncio.sleep(1)
return "Step 1 complete"
async def step2():
await asyncio.sleep(1)
return "Step 2 complete"
async def main():
result1 = await step1()
result2 = await step2()
print(result1)
print(result2)
asyncio.run(main())
Error Handling in Coroutines
Handle exceptions within coroutines using try-except blocks.
import asyncioasync def faulty_task():
try:
await asyncio.sleep(1)
raise ValueError("An error occurred")
except ValueError as e:
print(f"Error: {e}")
asyncio.run(faulty_task())
9. Error Handling in Asynchronous Code
Catching Exceptions
Use try-except blocks to catch exceptions in coroutines.
import asyncioasync def risky_task():
try:
await asyncio.sleep(1)
raise RuntimeError("Something went wrong")
except RuntimeError as e:
print(f"Handled error: {e}")
asyncio.run(risky_task())
Handling Task Failures
Check for task exceptions using Task.exception()
and Task.result()
.
import asyncioasync def fail_task():
await asyncio.sleep(1)
raise ValueError("Task failed")
async def main():
task = asyncio.create_task(fail_task())
await asyncio.sleep(2)
if task.exception():
print(f"Task raised an exception: {task.exception()}")
asyncio.run(main())
10. Synchronization Primitives
Using Locks
asyncio.Lock
is used to synchronize access to shared resources.
import asynciolock = asyncio.Lock()
async def critical_section():
async with lock:
print("Entered critical section")
await asyncio.sleep(1)
print("Exited critical section")
async def main():
await asyncio.gather(critical_section(), critical_section())
asyncio.run(main())
Using Events
asyncio.Event
is used for signaling between coroutines.
import asyncioevent = asyncio.Event()
async def waiter():
print("Waiting for event")
await event.wait()
print("Event triggered")
async def setter():
await asyncio.sleep(1)
event.set()
print("Event set")
async def main():
await asyncio.gather(waiter(), setter())
asyncio.run(main())
11. Using asyncio
with Third-Party Libraries
aiohttp
for Asynchronous HTTP
aiohttp
provides asynchronous HTTP client and server functionality. Install it with pip install aiohttp
.
Asynchronous HTTP Client:
import aiohttp
import asyncioasync def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
async def main():
html = await fetch('https://www.example.com')
print(html)
asyncio.run(main())
Asynchronous HTTP Server:
from aiohttp import webasync def handle(request):
return web.Response(text="Hello, Aiohttp")
app = web.Application()
app.router.add_get('/', handle)
if __name__ == "__main__":
web.run_app(app)
aiomysql
for Asynchronous MySQL
aiomysql
provides asynchronous MySQL support. Install it with pip install aiomysql
.
import aiomysql
import asyncioasync def fetch_data():
conn = await aiomysql.connect(host='127.0.0.1', port=3306, user='root', password='password', db='testdb')
async with conn.cursor() as cur:
await cur.execute("SELECT * FROM test_table")
result = await cur.fetchall()
print(result)
conn.close()
asyncio.run(fetch_data())
12. Best Practices and Optimization
Write Efficient Asynchronous Code
- Minimize Blocking Operations: Avoid blocking calls within asynchronous code.
- Use
await
Properly: Ensure thatawait
is used for I/O operations to avoid blocking the event loop.
Optimize Task Management
- Limit Concurrent Tasks: Control the number of concurrent tasks to avoid overwhelming the system.
- Use Task Queues: Manage tasks using queues to balance load.
Resource Management
- Close Resources: Ensure that resources (e.g., connections) are properly closed after use.
- Avoid Resource Leaks: Monitor and manage resource usage to prevent leaks.
13. Debugging and Testing
Debugging Asynchronous Code
- Use Logging: Implement logging to track coroutine execution and issues.
- Use Debugging Tools: Utilize tools like
pdb
andipdb
for interactive debugging.
Testing Asynchronous Code
- Use
pytest-asyncio
: A plugin forpytest
that provides support for testing asynchronous code.
import pytest
import asyncio
async def test_example():
assert await asyncio.sleep(1, result=42) == 42
14. Real-World Examples
Web Scraping with asyncio
and aiohttp
Asynchronous web scraping allows for efficient data extraction from multiple web pages.
import aiohttp
import asyncio
from bs4 import BeautifulSoupasync def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
async def parse(url):
html = await fetch(url)
soup = BeautifulSoup(html, 'html.parser')
print(soup.title.text)
async def main():
urls = ['https://www.example.com', 'https://www.example.org']
await asyncio.gather(*(parse(url) for url in urls))
asyncio.run(main())
Building a Chat Server
An asynchronous chat server can handle multiple clients concurrently.
import asyncioclients = []
async def handle_client(reader, writer):
clients.append(writer)
while True:
data = await reader.read(100)
if not data:
clients.remove(writer)
break
message = data.decode()
for client in clients:
if client != writer:
client.write(data)
await client.drain()
async def main():
server = await asyncio.start_server(handle_client, '127.0.0.1', 8888)
async with server:
await server.serve_forever()
asyncio.run(main())
15. Conclusion
asyncio
is a powerful library in Python for writing asynchronous code, enabling efficient handling of I/O-bound tasks and concurrent operations. By understanding core concepts such as the event loop, coroutines, and tasks, and applying best practices in code management and resource handling, you can leverage asyncio
to build responsive and scalable applications. Whether working with asynchronous I/O, network operations, or integrating with third-party libraries, asyncio
provides the tools and flexibility needed to achieve robust asynchronous programming in Python.