Mastering Java Programming for Engineering Applications: A Comprehensive Guide
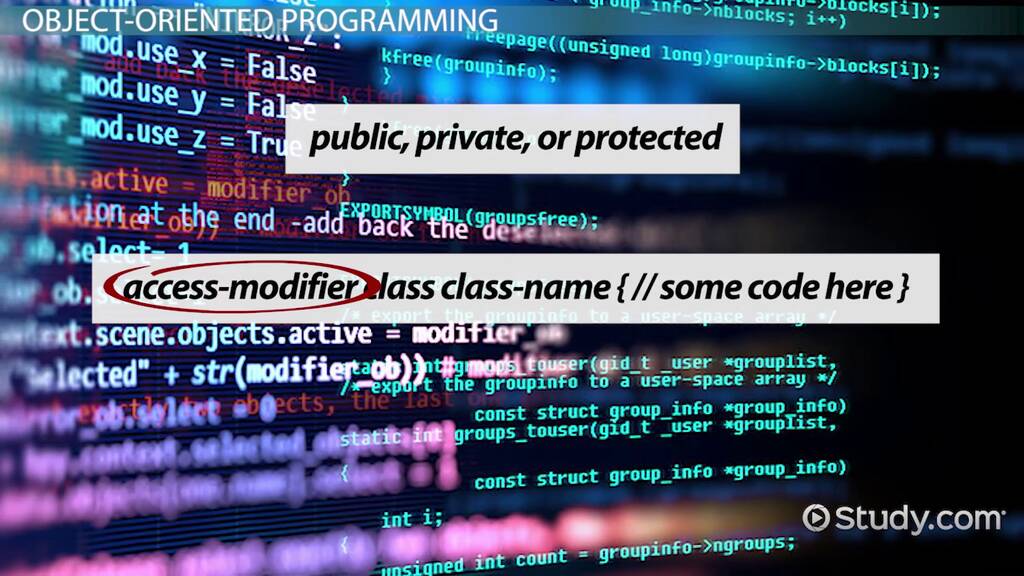
Introduction: Java is a versatile and widely-used programming language known for its platform independence, object-oriented programming (OOP) paradigm, and rich ecosystem of libraries and frameworks. With its robust features and extensive community support, Java is an ideal choice for developing engineering applications across various domains, including robotics, automation, simulation, and data analysis. In this comprehensive guide, we will explore the intricacies of programming in Java for engineering applications, covering everything from fundamental concepts and best practices to advanced techniques and real-world examples.
Section 1: Introduction to Java Programming for Engineers 1.1 Overview of Java: Java is a high-level, class-based programming language developed by Sun Microsystems (now owned by Oracle Corporation) in 1995. It is designed to be platform-independent, meaning that Java programs can run on any device that has a Java Virtual Machine (JVM) installed, making it ideal for cross-platform development.
1.2 Importance of Java in Engineering: Java offers several advantages for engineering applications, including:
- Platform Independence: Java’s “write once, run anywhere” philosophy allows engineers to develop applications that can run on any operating system or hardware platform with a JVM.
- Object-Oriented Programming: Java’s OOP features, such as classes, objects, inheritance, and polymorphism, provide a modular and reusable approach to software design, making it easier to develop and maintain complex engineering systems.
- Rich Ecosystem: Java has a vast ecosystem of libraries, frameworks, and tools for various engineering tasks, including numerical computation, data analysis, simulation, and visualization.
Section 2: Getting Started with Java Programming 2.1 Setting Up the Development Environment: To start programming in Java, engineers need to set up a development environment that includes the Java Development Kit (JDK) and an Integrated Development Environment (IDE) such as Eclipse, IntelliJ IDEA, or NetBeans. Engineers can download and install the JDK from the official Java website and choose an IDE based on their preferences and requirements.
2.2 Writing Your First Java Program: Once the development environment is set up, engineers can write their first Java program, typically a “Hello, World!” application, to get acquainted with the syntax and structure of Java code. Engineers can use the IDE’s code editor to write Java code, compile it into bytecode, and run it using the JVM.
Section 3: Essential Concepts in Java Programming 3.1 Basic Syntax and Language Constructs: Java syntax is similar to C and C++, making it relatively easy for engineers familiar with these languages to transition to Java. Key language constructs in Java include variables, data types, operators, control flow statements (such as if-else, switch-case), loops (such as for, while, do-while), and methods.
3.2 Object-Oriented Programming (OOP) Principles: Java is a pure object-oriented programming language, meaning that everything in Java is an object. Engineers use classes and objects to model real-world entities, encapsulate data and behavior, and promote code reuse through inheritance and polymorphism. Understanding OOP principles such as encapsulation, inheritance, polymorphism, and abstraction is essential for effective Java programming.
Section 4: Advanced Java Programming Techniques 4.1 Exception Handling: Exception handling is a critical aspect of Java programming, allowing engineers to gracefully handle runtime errors and exceptions that may occur during program execution. Engineers use try-catch blocks to catch exceptions, handle them appropriately, and maintain the robustness and reliability of their applications.
4.2 Multithreading and Concurrency: Java provides built-in support for multithreading and concurrency, allowing engineers to develop concurrent and parallel applications that can perform multiple tasks simultaneously. Engineers use Java’s Thread class and Executor framework to create and manage threads, synchronize access to shared resources, and prevent race conditions and deadlocks.
Section 5: Java Libraries and Frameworks for Engineering Applications 5.1 JavaFX for Graphical User Interfaces (GUIs): JavaFX is a modern GUI toolkit for Java applications, providing rich visualization capabilities for engineering applications. Engineers use JavaFX to create interactive user interfaces, 2D and 3D graphics, charts, and animations, enhancing the usability and aesthetics of their applications.
5.2 Apache Commons Math for Numerical Computation: Apache Commons Math is a comprehensive library for numerical computation and mathematical analysis in Java. Engineers use Apache Commons Math to perform complex mathematical operations, such as linear algebra, optimization, interpolation, and statistical analysis, in their engineering applications.
Section 6: Real-World Applications and Case Studies 6.1 Robotics and Automation: Java is widely used in robotics and automation applications for developing control systems, sensor interfaces, and communication protocols. Engineers use Java to program robotic platforms, such as drones, industrial robots, and autonomous vehicles, leveraging its platform independence and real-time capabilities.
6.2 Data Analysis and Visualization: Java is employed in data analysis and visualization applications for processing, analyzing, and visualizing large datasets. Engineers use Java libraries and frameworks, such as Apache Spark, Apache Hadoop, and JavaFX, to perform data mining, machine learning, and interactive data visualization tasks in engineering domains such as predictive maintenance, quality control, and supply chain management.
Section 7: Best Practices and Optimization Strategies 7.1 Code Reusability and Modularity: To improve code maintainability and scalability, engineers should follow best practices for code reusability and modularity in Java programming. Engineers should design classes and methods that are reusable, modular, and loosely coupled, promoting code organization, readability, and extensibility.
7.2 Performance Optimization: Java applications can benefit from performance optimization techniques to improve execution speed, memory efficiency, and resource utilization. Engineers can optimize Java code by minimizing object creation, reducing garbage collection overhead, optimizing data structures and algorithms, and leveraging profiling tools to identify performance bottlenecks.
Section 8: Future Trends and Developments 8.1 Internet of Things (IoT) and Edge Computing: As the Internet of Things (IoT) continues to grow, Java is expected to play a significant role in developing IoT solutions and edge computing applications. Engineers may leverage Java’s platform independence and lightweight runtime environment to develop IoT devices, edge gateways, and real-time data processing systems for various engineering applications.
8.2 Artificial Intelligence and Machine Learning: Java is increasingly being used in artificial intelligence (AI) and machine learning (ML) applications, thanks to libraries and frameworks such as Deeplearning4j, Weka, and Apache Mahout. Engineers may use Java for training and deploying ML models, developing intelligent agents, and integrating AI capabilities into engineering systems for tasks such as predictive maintenance, anomaly detection, and optimization.
Conclusion: Java programming offers engineers a powerful and versatile platform for developing a wide range of engineering applications, from robotics and automation to data analysis and visualization. By mastering the fundamental concepts, advanced techniques, and best practices outlined in this guide, engineers can leverage Java’s strengths to create robust, scalable, and high-performance software solutions that address complex engineering challenges in today’s rapidly evolving technological landscape. With its extensive ecosystem of libraries, frameworks, and tools, Java continues to be a preferred choice for engineering professionals seeking to innovate, optimize, and transform engineering processes and systems across diverse industries and domains.