Mastering Android App Development with Kotlin: A Comprehensive Guide
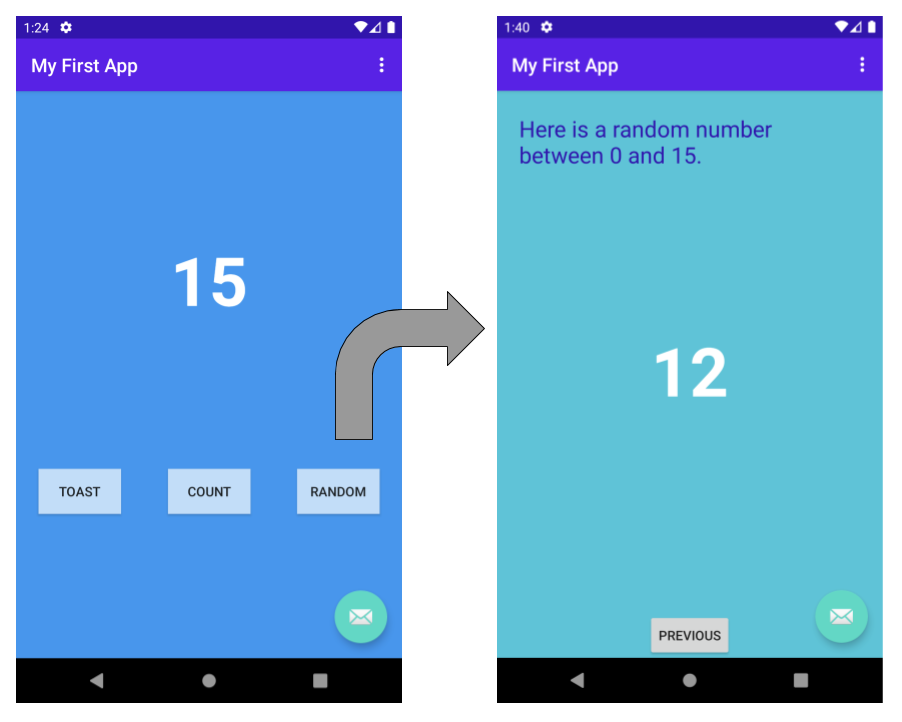
Introduction: Kotlin has emerged as a powerful programming language for Android app development, offering modern features, concise syntax, and seamless interoperability with Java. With its expressive and intuitive syntax, Kotlin enables developers to build robust and efficient Android applications while leveraging the full capabilities of the Android platform. In this comprehensive guide, we will explore the principles, methodologies, and best practices of programming in Kotlin for Android engineering applications, empowering developers to create high-quality and feature-rich Android apps.
Section 1: Introduction to Kotlin for Android Development
1.1 Overview of Kotlin Programming Language: Kotlin is a statically-typed programming language developed by JetBrains, designed to be fully interoperable with Java and run on the Java Virtual Machine (JVM). Kotlin offers concise syntax, null safety, type inference, extension functions, and other modern features that streamline Android app development and improve developer productivity.
1.2 Advantages of Kotlin for Android Development: Explore the advantages of using Kotlin for Android app development, including improved syntax, reduced boilerplate code, enhanced safety features, interoperability with Java libraries, and seamless integration with Android Studio. Learn how Kotlin’s features and capabilities enable developers to build scalable, maintainable, and performant Android applications.
Section 2: Getting Started with Kotlin for Android Development
2.1 Setting Up Development Environment: Set up your development environment for Kotlin Android development by installing Android Studio, configuring the Kotlin plugin, and creating a new Kotlin project. Learn how to set up build configurations, dependencies, and project structure for Android app development using Kotlin programming language.
2.2 Kotlin Syntax and Basics: Familiarize yourself with the syntax and basics of Kotlin programming language, including variables, data types, control flow statements, functions, and classes. Understand Kotlin’s syntax conventions, null safety features, type inference, and extension functions, and how they differ from Java syntax and conventions.
2.3 Interoperability with Java: Explore Kotlin’s interoperability with Java and learn how to seamlessly integrate Kotlin code with existing Java codebases, libraries, and frameworks. Understand how Kotlin’s interoperability features, such as Java-to-Kotlin conversion, Java interoperability annotations, and Java-friendly syntax, facilitate migration to Kotlin and collaboration between Kotlin and Java developers.
Section 3: Kotlin Programming Fundamentals for Android Development
3.1 UI Design and Layouts with Kotlin: Design user interfaces and layouts for Android apps using Kotlin programming language and XML layout files. Learn how to create UI elements, such as buttons, text views, images, and input fields, programmatically in Kotlin code, and how to define layout structures and styles using XML layout files.
3.2 Handling User Input and Events: Handle user input and events in Android apps using Kotlin programming language, including click events, touch events, and gesture events. Implement event listeners, callback functions, and event handling mechanisms in Kotlin code to respond to user interactions and trigger appropriate actions within the app.
3.3 Working with Views and ViewModels: Manipulate views, view properties, and view attributes programmatically in Kotlin code to dynamically update the user interface based on application logic and data changes. Implement ViewModels and LiveData objects using Kotlin’s architecture components to manage UI-related data and ensure separation of concerns in Android app development.
Section 4: Advanced Kotlin Techniques for Android Development
4.1 Asynchronous Programming with Coroutines: Implement asynchronous programming and concurrency in Android apps using Kotlin Coroutines, a lightweight and efficient concurrency framework. Learn how to use suspend functions, coroutine builders, and coroutine scopes to perform asynchronous tasks, network operations, and background processing in Android apps with Kotlin.
4.2 Data Persistence and Storage: Store and manage application data persistently in Android apps using Kotlin programming language and Android’s built-in data storage mechanisms. Explore SQLite databases, SharedPreferences, file I/O operations, and other data storage options in Android development with Kotlin, and learn how to implement data persistence strategies for efficient data management.
4.3 Networking and API Integration: Integrate with web services, RESTful APIs, and backend systems in Android apps using Kotlin programming language. Implement network requests, HTTP client libraries, and API communication protocols in Kotlin code to retrieve data, exchange information, and interact with remote servers and services in Android applications.
Section 5: Testing and Debugging Kotlin Android Apps
5.1 Unit Testing with Kotlin: Write unit tests for Android apps developed in Kotlin using frameworks such as JUnit and Mockito. Learn how to write test cases, mock dependencies, and verify behavior in Kotlin code to ensure code quality, reliability, and maintainability through automated testing.
5.2 Debugging and Error Handling: Debug and troubleshoot Kotlin Android apps using Android Studio’s debugging tools and Kotlin’s error handling mechanisms. Learn how to set breakpoints, inspect variables, and analyze stack traces to identify and resolve bugs, errors, and runtime exceptions in Kotlin code effectively.
5.3 Performance Optimization and Profiling: Optimize the performance of Kotlin Android apps using profiling tools and performance monitoring techniques. Analyze CPU usage, memory usage, network traffic, and battery consumption using Android Studio’s profiling tools and third-party performance monitoring libraries to identify bottlenecks, optimize resource usage, and improve app responsiveness and efficiency.
Section 6: Deploying and Publishing Kotlin Android Apps
6.1 Building and Packaging Android Apps: Build, package, and generate APK files for Kotlin Android apps using Android Studio’s build tools and Gradle build system. Configure build variants, signing configurations, and release settings to prepare Android apps for deployment and distribution on the Google Play Store and other app stores.
6.2 Testing and Quality Assurance: Conduct testing and quality assurance activities for Kotlin Android apps to ensure compatibility, functionality, and performance across different devices and Android versions. Perform device testing, compatibility testing, and beta testing using emulators, physical devices, and testing frameworks to identify and resolve issues before app release.
6.3 Publishing Android Apps on Google Play Store: Publish Kotlin Android apps on the Google Play Store and make them available to users worldwide. Prepare app listings, metadata, screenshots, and promotional materials for app store submission, and follow Google Play Store’s guidelines and policies for app publishing, distribution, and monetization.
Conclusion: Programming in Kotlin for Android engineering applications offers developers a powerful and versatile platform for building high-quality and feature-rich Android apps. By mastering the principles, methodologies, and best practices outlined in this guide, developers can leverage Kotlin’s modern features, syntax, and tools to create robust, efficient, and scalable Android applications that meet the needs of users and businesses. With proper training, collaboration, and adherence to industry standards, Kotlin empowers developers to innovate, iterate, and deliver compelling Android experiences that drive user engagement, satisfaction, and success in the dynamic world of Android app development.