Mastering Memory Management in Rust: Techniques, Ownership, and Lifetimes
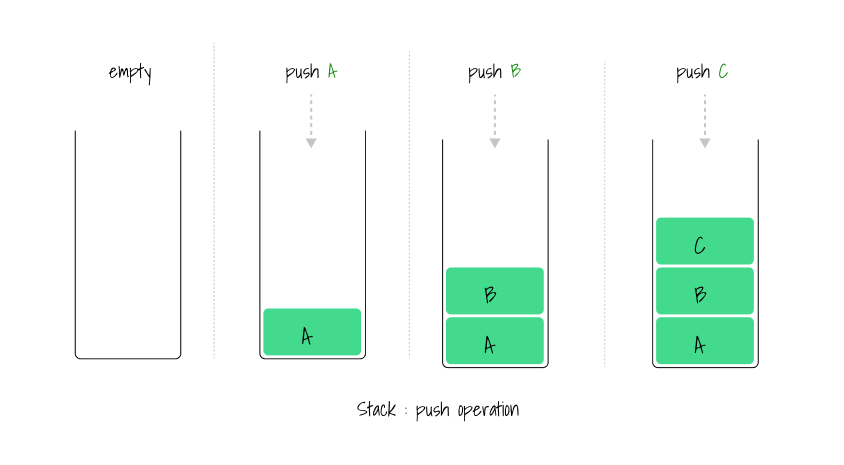
Introduction: Memory management is a critical aspect of programming, ensuring that resources are allocated and deallocated efficiently to prevent memory leaks and optimize performance. In Rust, a modern systems programming language, memory management is unique due to its ownership system, which guarantees memory safety without the need for a garbage collector. By understanding Rust’s memory management principles, including ownership, borrowing, and lifetimes, you can write safe, efficient, and reliable code. In this comprehensive guide, we’ll explore everything you need to know about managing memory in Rust, from basic concepts to advanced techniques and best practices.
- Understanding Ownership: In Rust, memory is managed through a system of ownership, which ensures that each piece of memory has a single owner responsible for deallocating it when it’s no longer needed. Ownership follows these key principles:
- Each value in Rust has a single owner.
- Values are dropped (deallocated) when they go out of scope.
- Ownership can be transferred, borrowed, or shared using Rust’s ownership rules.
For example, consider the following code snippet:
fn main() {
let s = String::from("hello"); // s is the owner of the String
println!("{}", s);
} // s goes out of scope and is dropped
- Borrowing and References: In addition to ownership, Rust provides borrowing and references as mechanisms for accessing and manipulating values without taking ownership. Borrowing allows multiple references to a value to exist simultaneously, subject to certain restrictions:
- Immutable references (&T) allow reading but not modifying the value.
- Mutable references (&mut T) allow both reading and modifying the value but only one mutable reference can exist at a time.
For example:
fn main() {
let mut s = String::from("hello");
modify_string(&mut s); // Pass a mutable reference to modify_string
println!("{}", s); // Print modified value
} fn modify_string(s: &mut String) {
s.push_str(", world!");
}
- Lifetimes and Borrow Checker: Rust’s borrow checker enforces memory safety by ensuring that references do not outlive the values they reference. This is achieved through the concept of lifetimes, which represent the scope for which a reference is valid. Lifetimes are denoted by apostrophes (‘), and the borrow checker analyzes code to ensure that references adhere to the lifetime rules.
For example:
fn main() {
let s;
{
let s1 = String::from("hello");
s = &s1; // Error: `s1` does not live long enough
} // `s1` goes out of scope
println!("{}", s);
}
- Memory Management Techniques: Rust provides several memory management techniques and features to handle complex scenarios and optimize performance, including:
- Ownership patterns: Use ownership patterns like move semantics, cloning, and borrowing to manage memory efficiently and prevent unnecessary allocations and deallocations.
- Lifetimes annotations: Specify lifetimes explicitly using lifetime annotations to clarify relationships between references and ensure memory safety.
- Unsafe code: Use the unsafe keyword to bypass Rust’s safety guarantees and perform low-level operations that require manual memory management, such as interacting with foreign functions or accessing hardware directly.
- Best Practices for Memory Management in Rust: To write safe, efficient, and idiomatic Rust code, consider following these best practices for memory management:
- Embrace Rust’s ownership model: Embrace Rust’s ownership, borrowing, and lifetimes system to write code that is memory-safe, thread-safe, and free of data races.
- Minimize unnecessary allocations: Minimize unnecessary allocations and deallocations by using references, borrowing, and ownership patterns to avoid unnecessary copying and cloning of values.
- Leverage Rust’s standard library: Leverage Rust’s standard library and built-in data structures, such as Vec, Option, and Result, which provide safe and efficient memory management mechanisms.
- Use unsafe code judiciously: Use unsafe code judiciously and only when necessary, ensuring that unsafe operations are properly encapsulated and thoroughly tested to minimize the risk of memory-related bugs and vulnerabilities.
- Conclusion: In conclusion, managing memory in Rust is a fundamental aspect of writing safe, efficient, and reliable code. By understanding Rust’s ownership, borrowing, and lifetimes system, mastering memory management techniques, and following best practices, you can harness the full power of Rust’s memory management capabilities. Whether you’re working on low-level systems programming, high-performance applications, or concurrent and parallel processing tasks, Rust provides the tools and features you need to manage memory safely and effectively. So dive into Rust’s memory management ecosystem, practice these techniques, and unlock the full potential of Rust for building robust and scalable software.