Mastering GUI Application Development with PyQt: A Comprehensive Guide
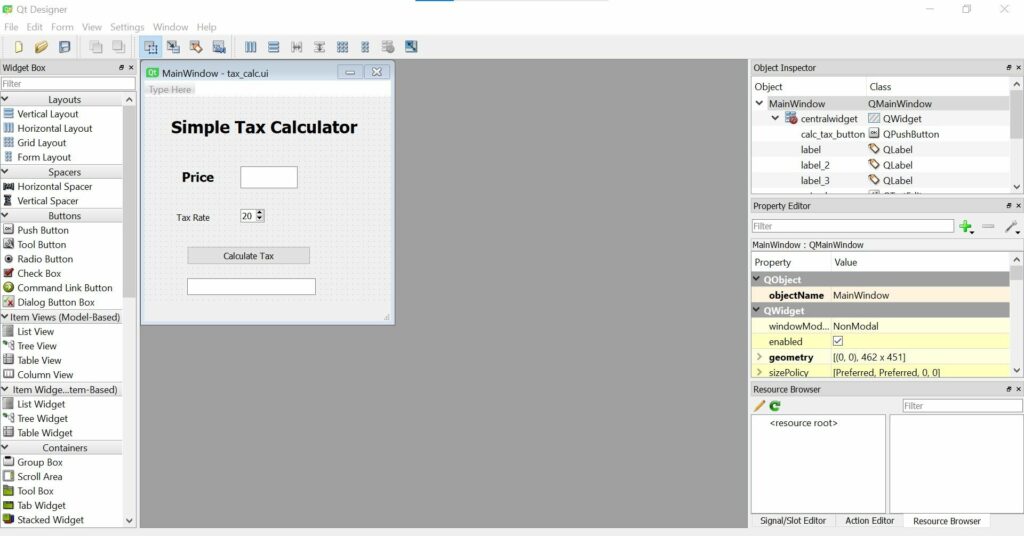
Introduction: GUI (Graphical User Interface) applications are ubiquitous in modern software development, providing users with intuitive interfaces for interacting with programs. PyQt, a Python binding for the Qt framework, offers a powerful toolkit for creating cross-platform GUI applications with Python. With PyQt, developers can leverage the extensive features of Qt to design sophisticated and visually appealing user interfaces. In this comprehensive guide, we’ll explore everything you need to know about creating GUI applications in PyQt, from setting up your development environment to designing complex interfaces and handling user interactions.
- Setting Up Your Development Environment: Before diving into PyQt development, it’s essential to set up your development environment. Here’s a step-by-step guide to getting started:
- Install Python: If you haven’t already, install Python on your system. PyQt supports Python 3.x, so make sure you have a compatible version installed.
- Install PyQt: Install PyQt using pip, the Python package manager. You can install PyQt5, the latest version of PyQt, by running the following command:
pip install PyQt5
- Install Qt Designer: Qt Designer is a graphical tool for designing Qt-based user interfaces. Install Qt Designer along with the Qt development tools from the Qt website.
- Introduction to PyQt: PyQt is a set of Python bindings for the Qt application framework, which allows developers to create cross-platform GUI applications with Python. PyQt provides access to the full range of Qt’s features, including widgets, layouts, signals and slots, and more. Here’s a simple PyQt program to get you started:
import sys
from PyQt5.QtWidgets import QApplication, QLabelapp = QApplication(sys.argv)
label = QLabel("Hello, PyQt!")
label.show()
sys.exit(app.exec_())
- Designing User Interfaces with Qt Designer: Qt Designer is a powerful tool for designing user interfaces visually. You can use Qt Designer to create layouts, add widgets, and customize the appearance of your application without writing any code. Here’s how to create a simple GUI application using Qt Designer:
- Open Qt Designer and create a new form.
- Drag and drop widgets from the toolbox onto the form.
- Arrange widgets and adjust their properties using the property editor.
- Save the form as a .ui file.
- Convert the .ui file to Python code using pyuic5, the PyQt UI compiler.
- Handling User Interactions: In PyQt, user interactions are handled using signals and slots, a mechanism for communication between objects. Signals are emitted when a particular event occurs, such as a button click or a mouse movement, while slots are functions that are executed in response to signals. Here’s an example of connecting a button click signal to a slot function:
from PyQt5.QtWidgets import QPushButton, QMessageBox, QVBoxLayout, QWidgetclass MyWidget(QWidget):
def __init__(self):
super().__init__()
layout = QVBoxLayout()
self.setLayout(layout)
button = QPushButton("Click Me")
button.clicked.connect(self.show_message)
layout.addWidget(button)
def show_message(self):
QMessageBox.information(self, "Message", "Button clicked!")
if __name__ == "__main__":
app = QApplication(sys.argv)
widget = MyWidget()
widget.show()
sys.exit(app.exec_())
- Deploying PyQt Applications: Once you’ve developed your PyQt application, you may want to deploy it to other users. PyQt applications can be packaged and distributed like any other Python application. You can use tools like PyInstaller or cx_Freeze to create standalone executables for Windows, macOS, and Linux platforms. Be sure to include the necessary PyQt dependencies when packaging your application.
- Best Practices for PyQt Development: To write efficient and maintainable PyQt code, consider following these best practices:
- Use layout managers: Use layout managers like QVBoxLayout, QHBoxLayout, and QGridLayout to create flexible and responsive user interfaces that adapt to different screen sizes and resolutions.
- Separate UI and logic: Separate the user interface code from the application logic to improve code organization and maintainability. Use the Model-View-Controller (MVC) or Model-View-ViewModel (MVVM) design patterns to achieve separation of concerns.
- Handle exceptions gracefully: Use try-except blocks to handle exceptions and errors gracefully, providing meaningful error messages to users and logging errors for debugging purposes.
- Follow Qt naming conventions: Follow Qt’s naming conventions for classes, methods, and variables to maintain consistency and readability in your codebase.
- Test your application: Write unit tests and integration tests to verify the functionality of your PyQt application and ensure that it behaves as expected under different conditions.
- Conclusion: In conclusion, PyQt is a powerful toolkit for creating cross-platform GUI applications with Python. By mastering PyQt’s features and techniques, you can design sophisticated and user-friendly interfaces for your applications. Whether you’re developing desktop utilities, multimedia applications, or productivity tools, PyQt provides the tools and flexibility you need to bring your ideas to life. So dive into PyQt development, experiment with different widgets and layouts, and create amazing GUI applications that delight users and enhance their productivity.