Mastering File Input/Output (I/O) Operations in Python: A Comprehensive Guide
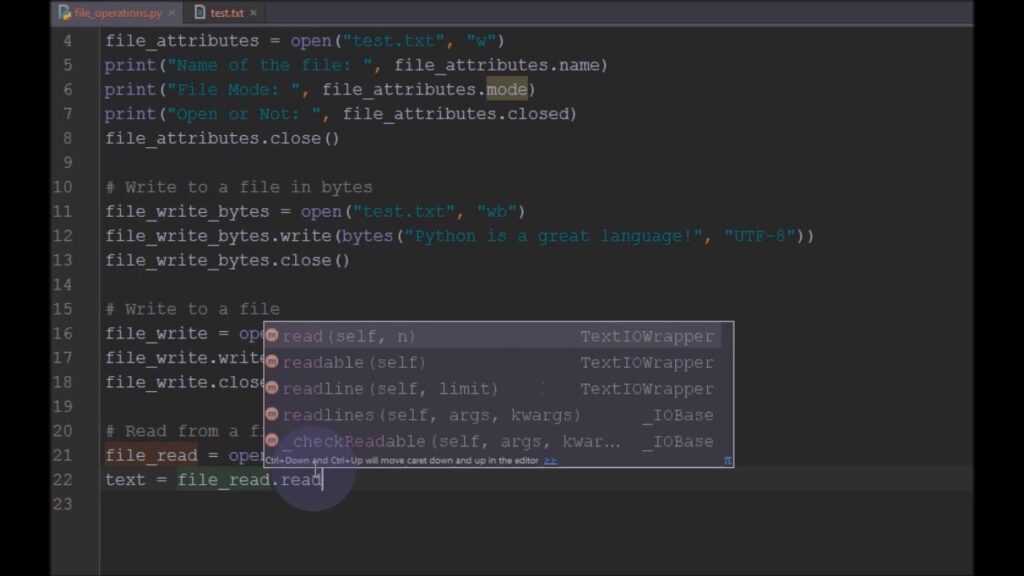
Introduction: File Input/Output (I/O) operations are fundamental tasks in programming, allowing you to read data from files, write data to files, and manipulate file contents. In Python, handling file I/O operations is straightforward and versatile, thanks to its built-in functions and libraries. Whether you’re reading data from a text file, writing output to a CSV file, or manipulating binary data, Python provides powerful tools and libraries to streamline the process. In this comprehensive guide, we’ll explore the various aspects of performing file I/O operations in Python, including reading and writing text files, working with different file modes, handling exceptions, and leveraging advanced techniques and libraries for more complex tasks.
- Reading and Writing Text Files: One of the most common file I/O operations in Python is reading and writing text files. Here’s how to perform these tasks:
- Reading from a text file:
with open('file.txt', 'r') as f:
data = f.read()
print(data)
- Writing to a text file:
with open('file.txt', 'w') as f:
f.write('Hello, World!')
- Working with File Modes: Python supports various file modes for opening files, allowing you to specify how the file should be opened and manipulated. Common file modes include:
- ‘r’: Read mode – Opens a file for reading only. The file pointer is placed at the beginning of the file.
- ‘w’: Write mode – Opens a file for writing only. If the file does not exist, it creates a new file. If the file exists, it truncates the file to zero length.
- ‘a’: Append mode – Opens a file for appending data to the end of the file. The file pointer is placed at the end of the file.
- ‘b’: Binary mode – Opens a file in binary mode, allowing you to read and write binary data.
- Handling Exceptions: When working with file I/O operations, it’s essential to handle exceptions to gracefully deal with potential errors. Common exceptions include FileNotFoundError, PermissionError, and IOError. Here’s an example of handling exceptions:
try:
with open('file.txt', 'r') as f:
data = f.read()
print(data)
except FileNotFoundError:
print("File not found.")
except PermissionError:
print("Permission denied.")
except IOError:
print("Error reading file.")
- Advanced Techniques and Libraries: Python offers several advanced techniques and libraries for performing file I/O operations, including:
- Using the csv module for reading and writing CSV files.
- Leveraging the json module for working with JSON data.
- Working with binary files using the struct module.
- Parsing XML and HTML files using libraries like xml.etree.ElementTree and BeautifulSoup.
- Best Practices: To ensure efficient and reliable file I/O operations in Python, consider the following best practices:
- Use context managers (with statement) to automatically close files after use.
- Always handle exceptions to gracefully deal with errors.
- Use meaningful variable names and comments to improve code readability.
- Test your code thoroughly, especially when working with external files or data.
- Conclusion: In conclusion, mastering file I/O operations is essential for any Python programmer, allowing you to read, write, and manipulate data stored in files. By understanding the basics of file I/O, working with different file modes, handling exceptions, and leveraging advanced techniques and libraries, you can perform a wide range of file operations efficiently and effectively. Whether you’re working with text files, CSV files, JSON data, or binary files, Python provides powerful tools and libraries to streamline the process. So practice these techniques, explore the available libraries, and incorporate file I/O operations into your Python projects to unlock their full potential for data manipulation and processing.